Struggling with unwieldy component state and performance issues in your React project? Mastering React Hooks can make a significant difference.
By following hook rules, breaking down state, and managing effect dependencies, you can streamline code and boost performance.
But there’s more—strategies like memoization, custom hooks, and linting can elevate your applications.
Ready to ensure your code is both maintainable and efficient? Let’s dive into these seven easy tips.
Understand Hook Rules
To use React Hooks effectively, you need to understand and follow their fundamental rules. First, only call Hooks at the top level of your function. This means you should avoid calling them inside loops, conditions, or nested functions. Doing so guarantees that Hooks are called in the same order each time a component renders, which is essential for React to correctly preserve the state.
Second, only call Hooks from React function components or custom Hooks. This rule guarantees that all stateful logic is clearly defined and easily trackable within your component’s scope.
Use State Wisely
Managing state effectively is crucial for maintaining performance and readability in your React components.
Start by breaking down your state into smaller, more manageable pieces. Use multiple useState
hooks instead of a single state object to keep your state updates more predictable and efficient.
Always initialize state with meaningful default values to prevent unexpected behaviors.
Effect Dependencies
Understanding effect dependencies is crucial for ensuring your useEffect
hooks run as expected and avoid unnecessary re-renders. Dependencies in useEffect
are values that the effect relies on. When one of these values changes, the effect runs again. You specify dependencies in an array as the second argument of useEffect
.
Failing to include the right dependencies can cause bugs or performance issues. As an example, if you fetch data inside useEffect
, you should include any state or props that trigger a new fetch. This guarantees the fetch runs when those values change.
Conversely, if you include too many dependencies, your effect might run more often than needed, resulting in inefficiency. Always review and optimize your dependency arrays for better performance.
Memoization
Memoization helps you optimize your React components by storing the results of expensive function calls and reusing them when the same inputs occur. This technique can vastly improve performance, especially in complex applications.
In React, you can use useMemo
and useCallback
hooks to achieve memoization.
useMemo
: Memoizes the result of a function computation.
useCallback
: Memoizes the actual function definition.
Dependency Array: Verify dependencies are correctly listed to avoid stale values.
Performance: Only use memoization when performance issues arise, as it adds complexity.
Testing: Regularly test to confirm memoization is improving performance.
Custom Hooks
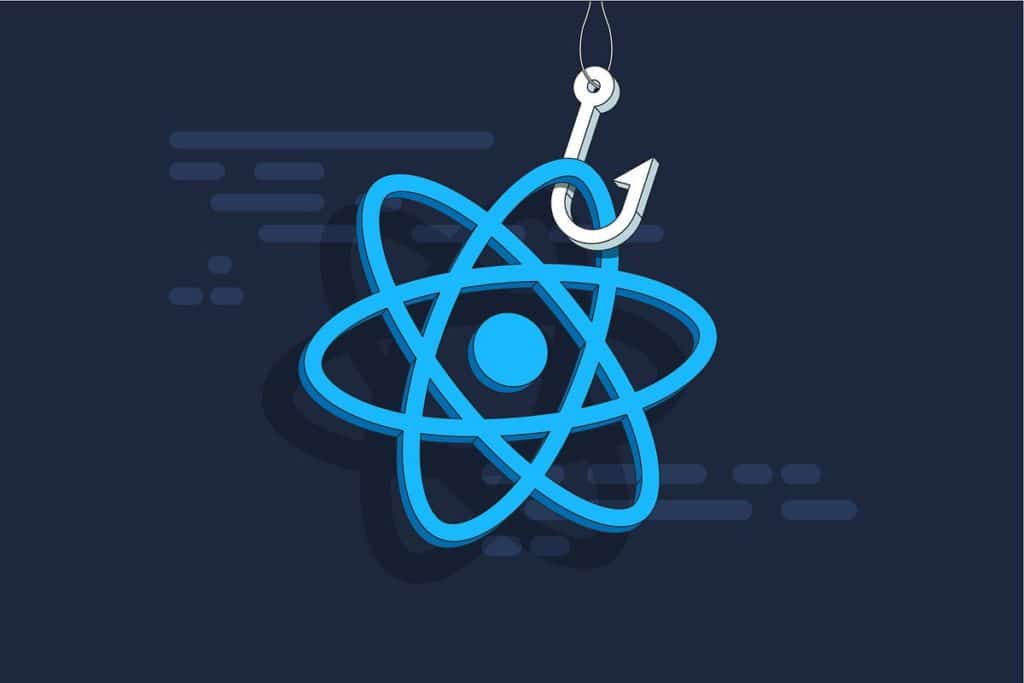
Custom Hooks let you extract and reuse stateful logic in your React components, making your code more modular and readable. By creating custom hooks, you can encapsulate logic that’s used by multiple components, avoiding duplication and making your code easier to maintain.
To create a custom hook, simply define a function that uses built-in hooks like useState
or useEffect
and export it. Use a naming convention like use
followed by a descriptive name to indicate it’s a hook, such as useFetchData
.
When you use your custom hook in a component, it behaves just like any other hook, managing state and side effects seamlessly. This leads to cleaner, more organized code and enhances reusability across your application.
Effect Cleanup
When working with React hooks, it’s vital to handle effect cleanup properly to prevent memory leaks and guarantee efficient performance. Cleanup guarantees that your component doesn’t hold onto resources or subscriptions when they’re no longer needed. To achieve this, you should always return a cleanup function inside useEffect
.
Key points to ponder:
- Remove Event Listeners: Verify any added event listeners are removed.
- Clear Timers: Eliminate any intervals or timeouts to prevent unnecessary calls.
- Cancel Network Requests: Abort any ongoing fetch requests to save bandwidth.
- Unsubscribe from Subscriptions: Unsubscribe from data streams or observables.
- Reset State: Restore or reset state variables to their initial values.
Linting
Linting is an essential practice when working with React hooks. Linting helps you catch common errors and maintain code quality. It guarantees you’re following best practices and coding standards, which is paramount for hooks because of their specific rules.
ESLint, a popular linting tool, offers a plugin called eslint-plugin-react-hooks
designed for this purpose. This plugin warns you if you misuse hooks, like forgetting dependencies in useEffect
or breaking the rules of hooks.
Conclusion
By following these seven tips, you’ll master React Hooks with ease. Envision your code as a well-oiled machine, with each hook playing its part seamlessly.
Understanding hook rules, managing state, and cleaning up effects will keep your components running smoothly. Memoization and custom hooks will streamline your logic, and linting will catch any issues before they become problems.
Adopt these practices to write maintainable, performant, and reliable React applications.